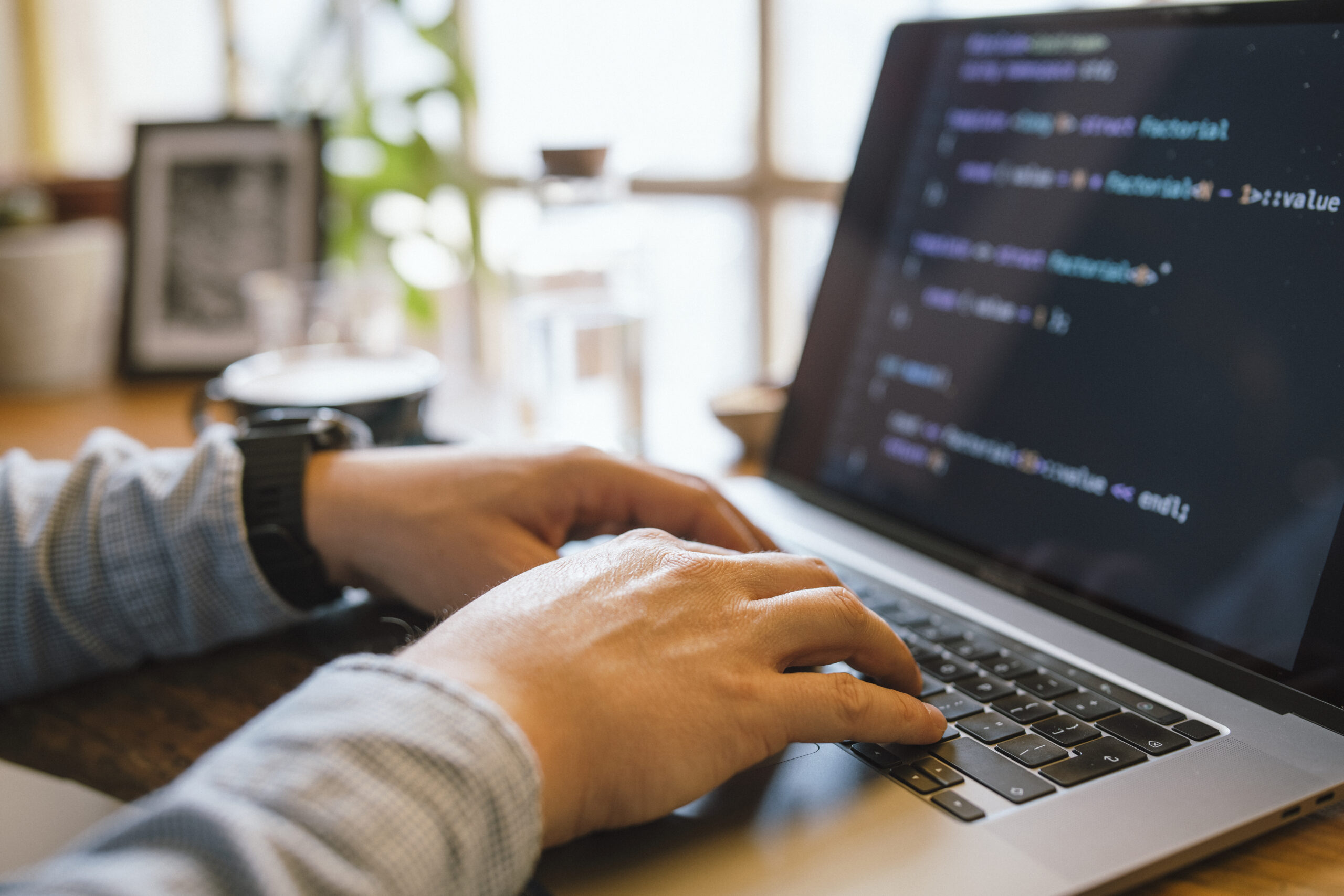
Debugging is one of the most crucial — nonetheless often disregarded — capabilities in a very developer’s toolkit. It isn't really just about fixing damaged code; it’s about comprehending how and why issues go Improper, and Finding out to Assume methodically to unravel challenges competently. Whether you are a starter or simply a seasoned developer, sharpening your debugging skills can save several hours of irritation and radically help your efficiency. Here's various tactics to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use daily. Whilst writing code is a person Component of advancement, understanding how to connect with it properly throughout execution is Similarly critical. Modern day development environments appear equipped with impressive debugging abilities — but numerous builders only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments enable you to set breakpoints, inspect the worth of variables at runtime, action via code line by line, and perhaps modify code to the fly. When employed correctly, they Enable you to observe exactly how your code behaves through execution, which can be a must have for monitoring down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-finish builders. They permit you to inspect the DOM, watch network requests, watch real-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can flip discouraging UI issues into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management over operating procedures and memory administration. Learning these resources could possibly have a steeper Discovering curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Regulate units like Git to know code historical past, uncover the precise instant bugs were being introduced, and isolate problematic variations.
Ultimately, mastering your resources implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem to make sure that when issues arise, you’re not lost in the dark. The better you know your tools, the greater time you can spend resolving the particular challenge in lieu of fumbling by the method.
Reproduce the challenge
The most vital — and often ignored — steps in effective debugging is reproducing the condition. Right before leaping to the code or creating guesses, builders will need to make a steady atmosphere or scenario wherever the bug reliably appears. With out reproducibility, fixing a bug will become a match of likelihood, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Talk to inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact disorders less than which the bug happens.
Once you’ve collected enough data, try to recreate the situation in your local natural environment. This could indicate inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting conditions or state transitions included. These checks not just enable expose the problem but in addition avoid regressions Down the road.
Sometimes, The problem can be environment-certain — it would materialize only on particular working devices, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications more effectively, check possible fixes securely, and communicate much more clearly together with your group or customers. It turns an abstract criticism right into a concrete challenge — and that’s where builders prosper.
Examine and Fully grasp the Error Messages
Error messages tend to be the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders must discover to treat mistake messages as direct communications in the system. They normally inform you what exactly occurred, where it transpired, and often even why it occurred — if you know the way to interpret them.
Start out by reading through the message thoroughly and in full. Quite a few developers, specially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — go through and understand them initially.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line variety? What module or function activated it? These questions can information your investigation and point you toward the dependable code.
It’s also useful to be aware of the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, assisting you pinpoint problems more rapidly, lower debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging levels include DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info during development, Facts for normal functions (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine difficulties, and FATAL in the event the process can’t proceed.
Steer clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, condition adjustments, enter/output values, and significant selection details with your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in creation environments where by stepping by means of code isn’t possible.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you may reduce the time it requires to identify issues, obtain further visibility into your here applications, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical job—it's a sort of investigation. To correctly determine and resolve bugs, developers ought to solution the procedure like a detective solving a mystery. This attitude will help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start off by accumulating proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect just as much applicable information as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a clear image of what’s happening.
Following, kind hypotheses. Request oneself: What might be creating this behavior? Have any variations a short while ago been designed on the codebase? Has this concern occurred before less than very similar conditions? The objective is to slender down opportunities and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge inside a managed setting. Should you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and Enable the final results direct you closer to the reality.
Spend shut focus to small facts. Bugs usually disguise while in the least predicted places—just like a missing semicolon, an off-by-just one error, or maybe a race situation. Be extensive and affected person, resisting the urge to patch The problem with out thoroughly comprehending it. Momentary fixes might cover the actual difficulty, just for it to resurface later.
And finally, continue to keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others comprehend your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in sophisticated devices.
Generate Tests
Creating exams is among the simplest tips on how to enhance your debugging expertise and Total progress performance. Checks not only assist catch bugs early but additionally serve as a safety Internet that provides you self confidence when building modifications in your codebase. A effectively-examined application is simpler to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with unit exams, which concentrate on personal functions or modules. These little, isolated tests can quickly expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a check fails, you instantly know exactly where to look, significantly lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—troubles that reappear right after previously being fastened.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assistance be sure that a variety of parts of your software operate with each other effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This volume of comprehension naturally qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a strong starting point. After the examination fails consistently, you'll be able to deal with fixing the bug and observe your take a look at pass when the issue is solved. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to become immersed in the situation—gazing your monitor for hours, trying Answer right after Resolution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you are also close to the code for as well extended, cognitive fatigue sets in. You may begin overlooking apparent errors or misreading code that you simply wrote just hours before. During this point out, your Mind turns into significantly less effective at issue-solving. A brief stroll, a coffee crack, or simply switching to a unique process for 10–15 minutes can refresh your aim. Quite a few developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You may perhaps out of the blue notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do something unrelated to code. It could feel counterintuitive, In particular under restricted deadlines, but it in fact causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a smart tactic. It gives your brain Place to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is really an opportunity to expand being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, each can instruct you a little something beneficial should you make the effort to replicate and review what went wrong.
Begin by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it are actually caught before with better practices like unit tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or understanding and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. After some time, you’ll begin to see designs—recurring problems or common issues—you could proactively prevent.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important aspects of your advancement journey. In fact, several of the best builders are not the ones who generate excellent code, but those who continually master from their blunders.
Eventually, Each and every bug you deal with adds a whole new layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to become superior at Anything you do.